The original game’s map “ground” consists of textures layered in a 2 dimensional tile map. I could extract the map from the game using MMExtensions, as described in my second post, however, the values of the tiles were ranging from 1 to 219. This suggests a total number of tiles of the original game is at least 219, or, some tiles are missing.
For simplicity’s sake, I decided to prepare a reduced tile map just for the partial remake, hence I had to pack the tiles. To reuse the tile map, I decided to reduce the tile ids to the smallest available ones, with the following relatively simple Python script.
from PIL import Image
import numpy
# Load the raw CSV
my_data = numpy.genfromtxt("emerald_tile_map.txt", delimiter=",")
print(my_data)
# Get the values - unique, since we're using a set
all_values = set()
for x in my_data:
for y in x:
if y not in all_values:
all_values.add(y)
sorted_vals = sorted(list(all_values))
print(f"total unique vals: {len(sorted_vals)}")
# Convert the values to the smallest available
# Set is sorted, but coming from C++, let's be sure
convert_map = {}
for i in range(len(sorted_vals)):
convert_map[sorted_vals[i]] = i
print(convert_map)
# Map the old values into new ones
new_data = numpy.copy(my_data)
for old, new in convert_map.items():
new_data[my_data == old] = new
print(new_data)
# Save the image
from PIL import Image
im = Image.fromarray(new_data)
im2 = im.convert("L")
im2.save("emerald_tile_map.bmp")
# Save to CSV
numpy.savetxt("emerald_tile_map2.csv", new_data, fmt="%d", delimiter=",")
The result is a map with tile values updated, for example: 1 -> 0, 174 -> 56, 218 -> 79 (80 values in total, ranging from 0 to 79 inclusive).
With the updated values, I could create a tile map. In my case, that was a 10×10 block of 128×128 tiles, with some missing. For the purpose I used Gimp, with a visible and snappy grid of 128px, and added all the necessary images, and eventually exported it to PNG. It looks like this:
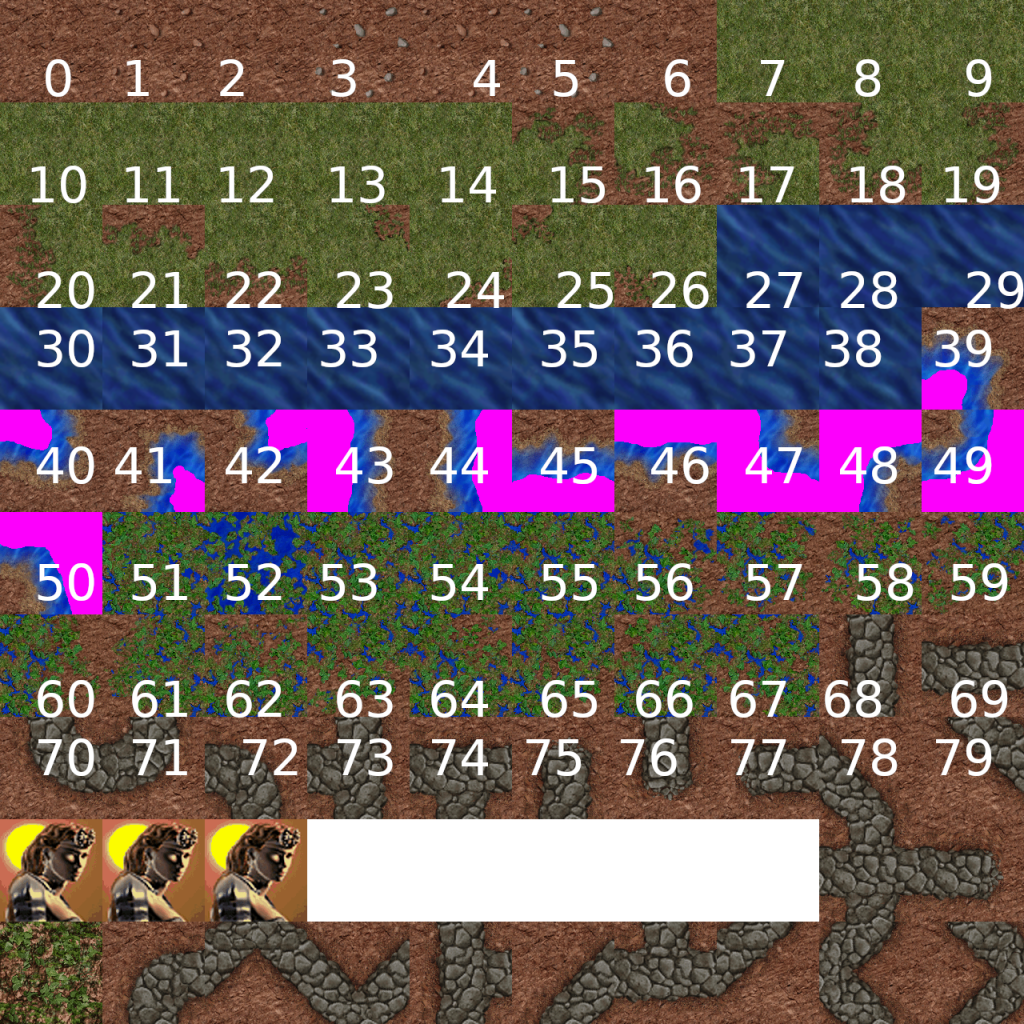
I have noticed some tiles seemed to require duplicating the original image, i.e. 7-14, looking at the map, all looked the same.
The PNG has temporarily added numbers, as those would be shown on the rendered 3d scene, for easier debugging:
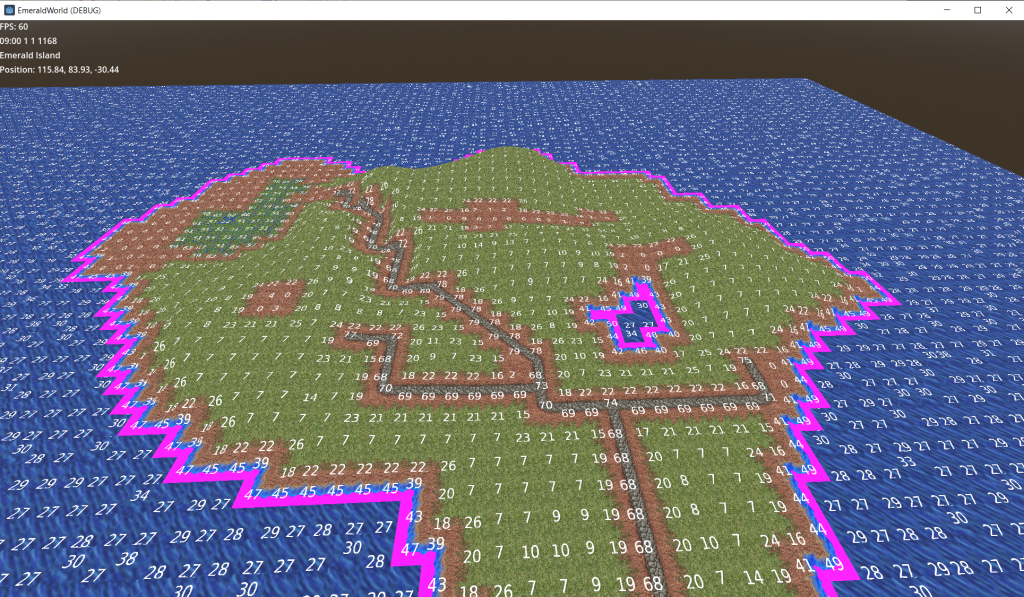
The result above is achieved with a fragment shader. The UV variable is an input in the range of [0,1], telling us what point of the texture is currently being sampled.
The texture we first look into is the tile map – to pick the id of the tile texture we need.
Given a tile id, we now must convert that to a row and column pair, but simple mod and div operations: we now have a “cell” in the 2d tile atlas.
All we need now, is to convert the UV position from meaning [0,1] on the entire map, to just look at a map’s single tile, and where relative to that we are. This is again normalized to [0,1]. As an example, consider the flow of the values we use.
The map has 128×128 cells. Let’s start with UV of [1/128 + 1/256, 1/128 + 1/256].
- This location on the tile map is the second row, and second column [1,1] (the area that maps to this is for both x and y: [1/128, 2/128]; the current point is in the middle of that). Let’s assume the tile ID ended up being 25.
- We are at 50% of the tile from the tile’s beginning to its end, hence mod_uv is [0.5, 0.5].
- We now must move to the tile id position on the atlas of the tile ID 25. Since the atlas in this example is 10×10 tiles, we select the 3rd row (values 20-29) and 6th column (counting from 20, “from zero”).
- We now just move by the [0.5, 0,5] vector from the start of the tile on the atlas, and voila.
More complex write-up than I wish, but here’s the code!
shader_type spatial;
// TODO understand and pick those:
//render_mode blend_mix,depth_draw_opaque,cull_back,diffuse_burley,specular_schlick_ggx;
uniform sampler2D atlas_texture_albedo : source_color,filter_nearest,repeat_enable;
uniform float atlas_tile_count;
uniform sampler2D tile_map;
uniform float tile_map_size;
uniform sampler2D height_map;
uniform float height_ratio = 50.0;
void vertex() {
VERTEX.y += texture(height_map, UV).r * height_ratio;
}
void fragment() {
vec2 base_uv = UV;
// Get the current tile id
float tile_id = texelFetch(tile_map, ivec2(base_uv * vec2(tile_map_size, tile_map_size)), 0).r * 255.0;
// Find the coords of the current tile
float atlas_col = mod(tile_id, atlas_tile_count);
float atlas_row = (tile_id - atlas_col) / atlas_tile_count;
// Normalize to [0,1] again, but in terms of a single tile
float tile_map_multiplier = 1.0 / tile_map_size;
vec2 mod_uv = mod(base_uv, tile_map_multiplier) / tile_map_multiplier;
// Get the UV position relative to an atlas tile size
float atlas_multiplier = 1.0 / atlas_tile_count;
vec2 atlas_uv = vec2(atlas_col, atlas_row) * atlas_multiplier + mod_uv * atlas_multiplier;
ALBEDO = texture(atlas_texture_albedo, atlas_uv).rgb;
}
Finally, here’s a very brief video showcasing the current state.